Now user need to create the Stub. In order to create the stub initially create the
NotifierStub Java Class while creating the class, implement the interface with the class.
In order to implements the interface click on the add button provided, the process as shown in below image.
Now the window will appear named as
Implement Interface Selection and type the interface name and proceed by clicking
OK button.
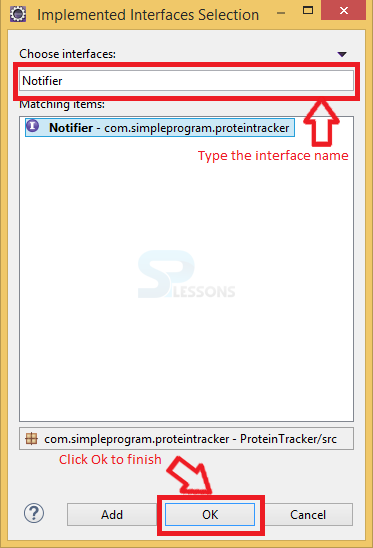
The code below demonstrate the new created class NotifierStub.
[c]package com.simpleprogram.proteintracker;
public class NotifierStub implements Notifier {
@Override
public boolean send(String message) {
return true;
}
[/c]
In order to use this class user make some changes to the TrackingService class as shown in below code.
[c]package com.simpleprogram.proteintracker;
import java.util.*;
public class TrackingService {
private int total;
private int goal;
private List <HistoryItem> history = new ArrayList<HistoryItem>();
private int historyId = 0;
private Notifier notifier;
public TrackingService(Notifier notifier)
{
this.notifier = notifier;
}
public void addProtein(int amount)
{
total +=amount;
history.add(new HistoryItem(historyId++, amount, "add", total));
if(total > goal)
{
boolean sendResult = notifier.send("goal met");
String historyMessage = "sent:goal met";
if(!sendRsult)
historyMessage = "send_error:goal met";
history.add(new HistoryItem(historyId++, 0, historyMessage, total));
}
{
boolean sendResult = notifier.send("goal met");
String historyMessage = "sent:goal met";
if(!sendResult)
historyMessage = "sent:goal met";
history.add(new HistoryItem(historyId++, 0, historyMssage, total));
}
}
public void removeProtein(int amount)
{
total -= amount;
if(total < 1);
total=0;
history.add(new HistoryItem(historyId++, amount, "subtract", total));
}
public int getTotal() {
return total;
}
public void setGoal(int value) throws InvalidException {
//if (value < 0) //throw new InvalidException(); goal = value; } public boolean isGoalMet(){ return total >= goal;
}
public List<HistoryItem> getHistory() {
return history;
}
}
[/c]
The Eclipse installed with the InfiniTest it will automatic run the test and get the output.