[html]
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>jQuery Mobile : Touch Event - Tap </title>
<meta name="viewport" content="width=device-width, minimum-scale=1.0, maximum-scale=1.0;">
<link rel="stylesheet" href="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.css" />
<script src="http://code.jquery.com/jquery-2.1.3.js"></script>
<script src="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.js"></script>
<script>
$(document).on("pagecreate", "#demoPage", function(){
$("li").on("tap",function() // bind on method with tap event to li
{
$(this).css("font-size", "30px"); // increase fontsize on tap
});
});
</script>
</head>
<body>
<div data-role="page" id="demoPage">
<div data-role="header">
<h1>Header (Default)</h1>
</div>
<!-- List items -->
<ul>
<li>USA</li>
<li>China</li>
<li>India</li>
<li>Japan</li>
<li>Germany</li>
</ul>
<div data-role="footer"> <!-- footer -->
<h3>Footer (Default)</h3>
</div>
</div>
</body>
</html>
[/html]
Output: When tapped on any of the list, the output will appear as follows.
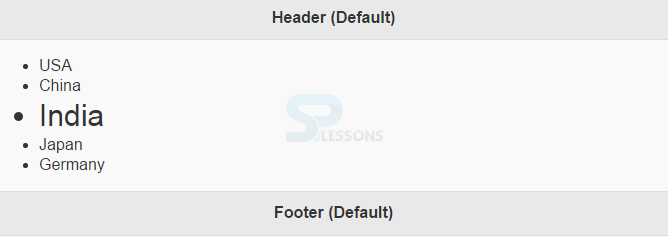
[html]
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>jQuery Mobile : Touch Event - Taphold </title>
<meta name="viewport" content="width=device-width, minimum-scale=1.0, maximum-scale=1.0;">
<link rel="stylesheet" href="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.css" />
<script src="http://code.jquery.com/jquery-2.1.3.js"></script>
<script src="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.js"></script>
<script>
$(document).on("pagecreate", "#demoPage", function(){
$("li").on("taphold",function() // bind on method with taphold event to li
{
$(this).css("font-size", "30px"); // increase fontsize on tap
});
});
</script>
</head>
<body>
<div data-role="page" id="demoPage">
<div data-role="header">
<h1>Header (Default)</h1>
</div>
<!-- List items -->
<ul>
<li>USA</li>
<li>China</li>
<li>India</li>
<li>Japan</li>
<li>Germany</li>
</ul>
<small>NOTE : Touch and hold on the List Items for approx 1 sec </small>
<div data-role="footer"> <!-- footer -->
<h3>Footer (Default)</h3>
</div>
</div>
</body>
</html>
[/html]
Output: When tap and holded on any of the list, the output will appear as follows.
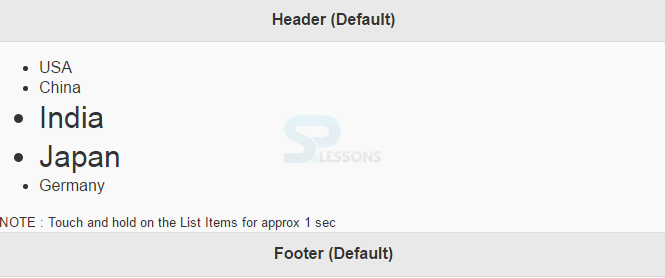
[html]
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>jQuery Mobile : Touch Event - Swipe </title>
<meta name="viewport" content="width=device-width, minimum-scale=1.0, maximum-scale=1.0;">
<link rel="stylesheet" href="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.css" />
<script src="http://code.jquery.com/jquery-2.1.3.js"></script>
<script src="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.js"></script>
<script>
$(document).on("pagecreate", "#demoPage", function(){
$(".myBox").on("swipe",function() // // swipe event binded to class .myBox
{
$(".output").text("Swipe Event Triggered");
});
});
</script>
<style>
.myBox {width: 250px; height:250px;
border:3px solid #444; background:#44c765;}
.output{font-size:30px; color:#fa4b2a;}
</style>
</head>
<body>
<div data-role="page" id="demoPage">
<div data-role="header">
<h1>Touch Event - Swipe </h1>
</div>
<div class="myBox"> </div>
<p class="output"></p>
<small>NOTE : Swipe on the Box Horizontally or Vertically </small>
<div data-role="footer"> <!-- footer -->
<h3>Footer (Default)</h3>
</div>
</div>
</body>
</html>
[/html]
Output: When tap and holded on any of the list, the output will appear as follows.
Likewise, swipeleft and swiperight can be executed.