Initially, create a XML file with the root element
class
,
student
as the child and firstname, lastname, nickname and marks as the sub-childs.
[xml]
<?xml version="1.0"?>
<class>
<student rollno="393">
<firstname>John</firstname>
<lastname>Mike</lastname>
<nickname>Jom</nickname>
<marks>85</marks>
</student>
<student rollno="493">
<firstname>Rafeal</firstname>
<lastname>Nadal</lastname>
<nickname>Rafa</nickname>
<marks>95</marks>
</student>
<student rollno="593">
<firstname>Samuel</firstname>
<lastname>Johnson</lastname>
<nickname>Sam</nickname>
<marks>90</marks>
</student>
</class>
[/xml]
UserHandler.java
[java]
package com.splessons.xml;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
public class UserHandler extends DefaultHandler {
boolean bFirstName = false;
boolean bLastName = false;
boolean bNickName = false;
boolean bMarks = false;
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
if (qName.equalsIgnoreCase("student")) {
String rollNo = attributes.getValue("rollno");
System.out.println("Roll No : " + rollNo);
} else if (qName.equalsIgnoreCase("firstname")) {
bFirstName = true;
} else if (qName.equalsIgnoreCase("lastname")) {
bLastName = true;
} else if (qName.equalsIgnoreCase("nickname")) {
bNickName = true;
}
else if (qName.equalsIgnoreCase("marks")) {
bMarks = true;
}
}
@Override
public void endElement(String uri, String localName, String qName) throws SAXException {
if (qName.equalsIgnoreCase("student")) {
System.out.println("End Element :" + qName);
}
}
@Override
public void characters(char ch[],
int start, int length) throws SAXException {
if (bFirstName) {
System.out.println("First Name: "
+ new String(ch, start, length));
bFirstName = false;
} else if (bLastName) {
System.out.println("Last Name: "
+ new String(ch, start, length));
bLastName = false;
} else if (bNickName) {
System.out.println("Nick Name: "
+ new String(ch, start, length));
bNickName = false;
} else if (bMarks) {
System.out.println("Marks: "
+ new String(ch, start, length));
bMarks = false;
}
}
}
[/java]
SAXParserDemo.java : In the below code, package
org.xml.sax
defines SAX interfaces. this bundle characterizes handlerbase-a default execution of a base polish for different handlers.
[java]
package com.splessons.xml;
import java.io.File;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
public class SAXParserDemo {
public static void main(String[] args){
try {
File inputFile = new File("input.txt");
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser saxParser = factory.newSAXParser();
UserHandler userhandler = new UserHandler();
saxParser.parse(inputFile, userhandler);
} catch (Exception e) {
e.printStackTrace();
}
}
}
class UserHandler extends DefaultHandler {
boolean bFirstName = false;
boolean bLastName = false;
boolean bNickName = false;
boolean bMarks = false;
@Override
public void startElement(String uri,
String localName, String qName, Attributes attributes)
throws SAXException {
if (qName.equalsIgnoreCase("student")) {
String rollNo = attributes.getValue("rollno");
System.out.println("Roll No : " + rollNo);
} else if (qName.equalsIgnoreCase("firstname")) {
bFirstName = true;
} else if (qName.equalsIgnoreCase("lastname")) {
bLastName = true;
} else if (qName.equalsIgnoreCase("nickname")) {
bNickName = true;
}
else if (qName.equalsIgnoreCase("marks")) {
bMarks = true;
}
}
@Override
public void endElement(String uri,
String localName, String qName) throws SAXException {
if (qName.equalsIgnoreCase("student")) {
System.out.println("End Element :" + qName);
}
}
@Override
public void characters(char ch[],
int start, int length) throws SAXException {
if (bFirstName) {
System.out.println("First Name: "
+ new String(ch, start, length));
bFirstName = false;
} else if (bLastName) {
System.out.println("Last Name: "
+ new String(ch, start, length));
bLastName = false;
} else if (bNickName) {
System.out.println("Nick Name: "
+ new String(ch, start, length));
bNickName = false;
} else if (bMarks) {
System.out.println("Marks: "
+ new String(ch, start, length));
bMarks = false;
}
}
}
[/java]
In the above code,
=> package javax.xml.parsers characterizes saxparserfactory superbness which returns saxparser.
=> Saxparserfactory thing produces case of parser. parser interface characterizes the strategy like setdocumenthandler() to setup event handlers and parse() to parse record.
=> Documenthandler interface characterizes startdocument(), enddocument(), startelement(), endelement() and are conjured while xml tag is recognixed and characterizes characters() and summoned while experiences printed content inside the detail.
=> Errorhandler interface characterizes strategies like blunder(), fatalerror(), alert() and are conjured because of different parsing botches.
=> DTD handler interface characterizes methods that are conjured while preparing definitions in a dtd.
=> Entity resolver interface characterizes strategies like resolveentity() is conjured while the parser need to wind up mindful of the certainties related to the guide of a uri.
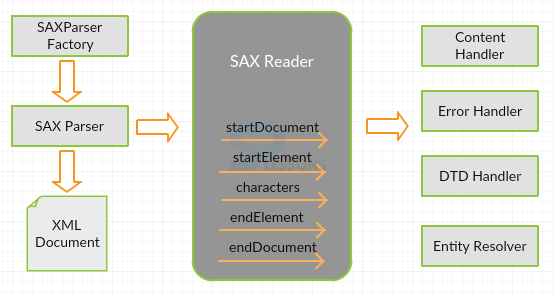
Output:
[java]
Roll No : 393
First Name: John
Last Name: Mike
Nick Name: Jom
Marks: 85
End Element :student
Roll No : 493
First Name: Rafeal
Last Name: Nadal
Nick Name: Rafa
Marks: 95
End Element :student
Roll No : 593
First Name: Samuel
Last Name: Johnson
Nick Name: Sam
Marks: 90
End Element :student
[/java]