Three.JS allow user to export individual geometry or entire scene. In order to export the geometry user need to reference to the
Geometry Exporter.js .
The code below demonstrates the Three.js Exporter methods app.js is shown below.
[c]
var example = (function() {
"use strict";
var scene = new THREE.Scene(),
light = new THREE.PointLight(0xffffff),
camera,
renderer = new THREE.WebGLRenderer(),
sphere;
function initScene() {
renderer.setSize(window.innerWidth, window.innerHeight);
document.getElementById("webgl-container").appendChild(renderer.domElement);
scene.add(light);
camera = new THREE.PerspectiveCamera(
35,
window.innerWidth / window.innerHeight,
1,
1000
);
camera.position.z = 80;
scene.add(camera);
var material = new THREE.MeshBasicMaterial({
color: 0x0000ff,
wireframe: true
});
sphere = new THREE.Mesh(
new THREE.SphereGeometry(
20,
10,
10),
material);
sphere.name = "sphere";
var exporter = new THREE.GeometryExporter();
var exportedSphereObject = exporter.parse(sphere.geometry);
var serializedExportedSphere = JSON.stringify(exportedSphereObject);
console.log(serializedExportedSphere);
console.log(JSON.stringify(serializedExportedSphere));
var loader = new THREE.JSONLoader();
var model = loader.parse(JSON.parse(serializedExportedSphere));
var mesh = new THREE.Mesh(model.geometry, material);
scene.add(mesh);
//var sceneExporter = new THREE.SceneExporter();
//var exportedSceneObject = sceneExporter.parse(scene);
render();
};
function render() {
renderer.render(scene, camera);
requestAnimationFrame(render);
};
window.onload = initScene;
return {
scene: scene,
sphere: sphere
}
})();
[/c]
The code below demonstrates the index.html file is shown.
[c]
<!DOCTYPE html>
<html>
<head>
<title>Intro to WebGL with three.js</title>
</head>
<body>
<div id="webgl-container"></div>
<script src="scripts/three.js"></script>
<script src="scripts/GeometryExporter.js"></script>
<!--<script src="scripts/SceneExporter.js"></script>-->
<script src="scripts/app.js"></script>
</body>
</html>
[/c]
Result
By running the above code in a preferred browser, user will get the output is as shown below.
In order to export the geometry user need to load the geometry into the work space and the tell the loader to parse JSON now create a new instance to the mesh which are passing in the geometry and any materials. Finally user can add these into setup now export the entire Three.js scene.
Three.js Editor
Three.js is nice visual editor for scenes and which can be quite useful for experiments. Three.JS editor available in the
Three.JS official web site. The image below demonstrates the Three.js editor is as shown below.
Now user can add the objects and some basic shapes into the editor by using the Add menu is shown in below image.
Now user can change the dimensions of the object by using scale option and user can also rotate the object and the change the position of the object which are shown in the below image.
Now user can export the the object by using available export options which are shown in below image.
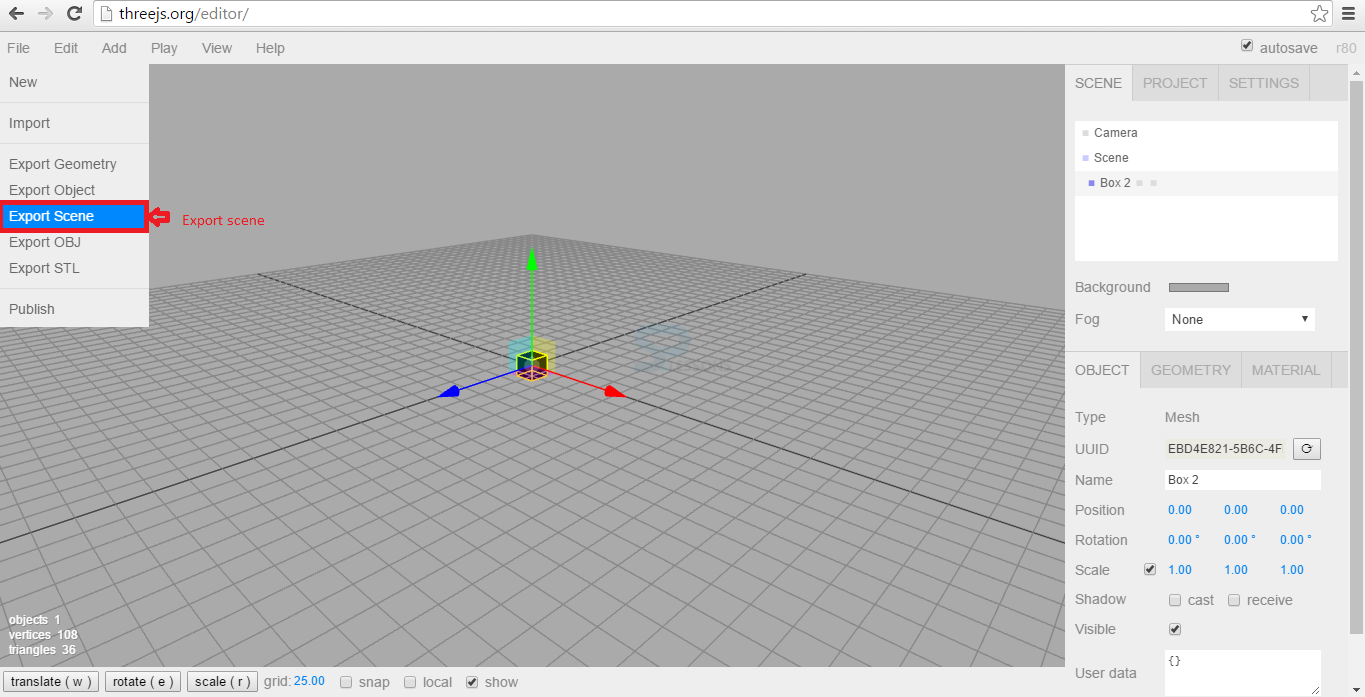
If user click on the Export scene then user can get the Exportr box code is as shoiwn below.
[c]
{
"metadata": {
"version": 4.4,
"type": "Object",
"generator": "Object3D.toJSON"
},
"geometries": [
{
"uuid": "D5153AB8-3672-4B54-A2B6-D3DDD12918AB",
"type": "BoxBufferGeometry",
"width": 1,
"height": 1,
"depth": 1
}],
"materials": [
{
"uuid": "77B7FD94-01DB-4906-962F-A4C91EA48343",
"type": "MeshStandardMaterial",
"color": 16777215,
"roughness": 0.5,
"metalness": 0.5,
"emissive": 0,
"depthFunc": 3,
"depthTest": true,
"depthWrite": true,
"skinning": false,
"morphTargets": false
}],
"object": {
"uuid": "12AEEA99-3D6E-458B-8E39-C7E73E3E5CAB",
"type": "Scene",
"name": "Scene",
"matrix": [1,0,0,0,0,1,0,0,0,0,1,0,0,0,0,1],
"children": [
{
"uuid": "2E0EC055-794A-43AF-9EB9-C0055E3C9573",
"type": "Mesh",
"name": "Box 1",
"matrix": [1,0,0,0,0,1,0,0,0,0,1,0,0,0,0,1],
"geometry": "D5153AB8-3672-4B54-A2B6-D3DDD12918AB",
"material": "77B7FD94-01DB-4906-962F-A4C91EA48343"
}],
"background": 11184810
}
}
[/c]