The following is an example of SOAP, before going to know about this example user need to know about WSDL why because SOAP uses WSDL. The WSDL is called as wiz-dull, where SOAP and UDDI uses WSDL protocol, the definitions of WSDL are used to describe web services and it is also a XML based registry.
The functionality of WSDL is how to authenticate a web service and to know the operations performed by the web services and WSDL used by SOAP to provide data over the network with the help of HTTP. This example will be depends on the following WSDL data link.
http://www.webservicex.com/globalweather.asmx?WSDL
To extract the data from the above file first create one folder with any name such as
test_wsdl
and under this folder create another folder with the name
src
. Now write the following command in the command prompt as follows.
Then another file will be created in the folder as follows which will have all the java files those need to be placed in eclipse.
The following is the structure of an application.
Test_wsdl.java
[java]package com.splessons;
import net.webservicex.GlobalWeather;
import net.webservicex.GlobalWeatherSoap;
public class Test_wsdl {
public static void main(String args[]){
GlobalWeather globalweather=new GlobalWeather();
GlobalWeatherSoap globalweathersoap=globalweather.getGlobalWeatherSoap();
String countryName=args[0];
String value=globalweathersoap.getCitiesByCountry(countryName);
System.out.println(value);
}
}
[/java]
GetCitiesByCountry.java
[java]
package net.webservicex;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"countryName"
})
@XmlRootElement(name = "GetCitiesByCountry")
public class GetCitiesByCountry {
@XmlElement(name = "CountryName")
protected String countryName;
public String getCountryName() {
return countryName;
}
public void setCountryName(String value) {
this.countryName = value;
}
}
[/java]
GetCitiesByCountryResponse.java
[java]
package net.webservicex;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"getCitiesByCountryResult"
})
@XmlRootElement(name = "GetCitiesByCountryResponse")
public class GetCitiesByCountryResponse {
@XmlElement(name = "GetCitiesByCountryResult")
protected String getCitiesByCountryResult;
public String getGetCitiesByCountryResult() {
return getCitiesByCountryResult;
}
public void setGetCitiesByCountryResult(String value) {
this.getCitiesByCountryResult = value;
}
}
[/java]
GetWeather.java
[java]
package net.webservicex;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"cityName",
"countryName"
})
@XmlRootElement(name = "GetWeather")
public class GetWeather {
@XmlElement(name = "CityName")
protected String cityName;
@XmlElement(name = "CountryName")
protected String countryName;
public String getCityName() {
return cityName;
}
public void setCityName(String value) {
this.cityName = value;
}
public String getCountryName() {
return countryName;
}
public void setCountryName(String value) {
this.countryName = value;
}
}
[/java]
GetWeatherResponse.java
[java]
package net.webservicex;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"getWeatherResult"
})
@XmlRootElement(name = "GetWeatherResponse")
public class GetWeatherResponse {
@XmlElement(name = "GetWeatherResult")
protected String getWeatherResult;
public String getGetWeatherResult() {
return getWeatherResult;
}
public void setGetWeatherResult(String value) {
this.getWeatherResult = value;
}
}
[/java]
GlobalWeather.java
[java]
package net.webservicex;
import java.net.MalformedURLException;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.ws.Service;
import javax.xml.ws.WebEndpoint;
import javax.xml.ws.WebServiceClient;
import javax.xml.ws.WebServiceException;
import javax.xml.ws.WebServiceFeature;
@WebServiceClient(name = "GlobalWeather", targetNamespace = "http://www.webserviceX.NET", wsdlLocation = "http://www.webservicex.com/globalweather.asmx?WSDL")
public class GlobalWeather
extends Service
{
private final static URL GLOBALWEATHER_WSDL_LOCATION;
private final static WebServiceException GLOBALWEATHER_EXCEPTION;
private final static QName GLOBALWEATHER_QNAME = new QName("http://www.webserviceX.NET", "GlobalWeather");
static {
URL url = null;
WebServiceException e = null;
try {
url = new URL("http://www.webservicex.com/globalweather.asmx?WSDL");
} catch (MalformedURLException ex) {
e = new WebServiceException(ex);
}
GLOBALWEATHER_WSDL_LOCATION = url;
GLOBALWEATHER_EXCEPTION = e;
}
public GlobalWeather() {
super(__getWsdlLocation(), GLOBALWEATHER_QNAME);
}
public GlobalWeather(WebServiceFeature... features) {
super(__getWsdlLocation(), GLOBALWEATHER_QNAME, features);
}
public GlobalWeather(URL wsdlLocation) {
super(wsdlLocation, GLOBALWEATHER_QNAME);
}
public GlobalWeather(URL wsdlLocation, WebServiceFeature... features) {
super(wsdlLocation, GLOBALWEATHER_QNAME, features);
}
public GlobalWeather(URL wsdlLocation, QName serviceName) {
super(wsdlLocation, serviceName);
}
public GlobalWeather(URL wsdlLocation, QName serviceName, WebServiceFeature... features) {
super(wsdlLocation, serviceName, features);
}
@WebEndpoint(name = "GlobalWeatherSoap")
public GlobalWeatherSoap getGlobalWeatherSoap() {
return super.getPort(new QName("http://www.webserviceX.NET", "GlobalWeatherSoap"), GlobalWeatherSoap.class);
}
@WebEndpoint(name = "GlobalWeatherSoap")
public GlobalWeatherSoap getGlobalWeatherSoap(WebServiceFeature... features) {
return super.getPort(new QName("http://www.webserviceX.NET", "GlobalWeatherSoap"), GlobalWeatherSoap.class, features);
}
private static URL __getWsdlLocation() {
if (GLOBALWEATHER_EXCEPTION!= null) {
throw GLOBALWEATHER_EXCEPTION;
}
return GLOBALWEATHER_WSDL_LOCATION;
}
}
[/java]
GlobalWeatherSoap.java
[java]
package net.webservicex;
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebResult;
import javax.jws.WebService;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.ws.RequestWrapper;
import javax.xml.ws.ResponseWrapper;
@WebService(name = "GlobalWeatherSoap", targetNamespace = "http://www.webserviceX.NET")
@XmlSeeAlso({
ObjectFactory.class
})
public interface GlobalWeatherSoap {
@WebMethod(operationName = "GetWeather", action = "http://www.webserviceX.NET/GetWeather")
@WebResult(name = "GetWeatherResult", targetNamespace = "http://www.webserviceX.NET")
@RequestWrapper(localName = "GetWeather", targetNamespace = "http://www.webserviceX.NET", className = "net.webservicex.GetWeather")
@ResponseWrapper(localName = "GetWeatherResponse", targetNamespace = "http://www.webserviceX.NET", className = "net.webservicex.GetWeatherResponse")
public String getWeather(
@WebParam(name = "CityName", targetNamespace = "http://www.webserviceX.NET")
String cityName,
@WebParam(name = "CountryName", targetNamespace = "http://www.webserviceX.NET")
String countryName);
@WebMethod(operationName = "GetCitiesByCountry", action = "http://www.webserviceX.NET/GetCitiesByCountry")
@WebResult(name = "GetCitiesByCountryResult", targetNamespace = "http://www.webserviceX.NET")
@RequestWrapper(localName = "GetCitiesByCountry", targetNamespace = "http://www.webserviceX.NET", className = "net.webservicex.GetCitiesByCountry")
@ResponseWrapper(localName = "GetCitiesByCountryResponse", targetNamespace = "http://www.webserviceX.NET", className = "net.webservicex.GetCitiesByCountryResponse")
public String getCitiesByCountry(
@WebParam(name = "CountryName", targetNamespace = "http://www.webserviceX.NET")
String countryName);
}
[/java]
ObjectFactory.java
[java]
package net.webservicex;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
@XmlRegistry
public class ObjectFactory {
private final static QName _String_QNAME = new QName("http://www.webserviceX.NET", "string");
public ObjectFactory() {
}
public GetWeather createGetWeather() {
return new GetWeather();
}
public GetCitiesByCountryResponse createGetCitiesByCountryResponse() {
return new GetCitiesByCountryResponse();
}
public GetWeatherResponse createGetWeatherResponse() {
return new GetWeatherResponse();
}
/**
* Create an instance of {@link GetCitiesByCountry }
*
*/
public GetCitiesByCountry createGetCitiesByCountry() {
return new GetCitiesByCountry();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.webserviceX.NET", name = "string")
public JAXBElement<String> createString(String value) {
return new JAXBElement<String>(_String_QNAME, String.class, null, value);
}
}
[/java]
package-info.java
[java]@javax.xml.bind.annotation.XmlSchema(namespace = "http://www.webserviceX.NET", elementFormDefault = javax.xml.bind.annotation.XmlNsForm.QUALIFIED)
package net.webservicex;
[/java]
Now add the arguments as follows by clicking on run configurations.
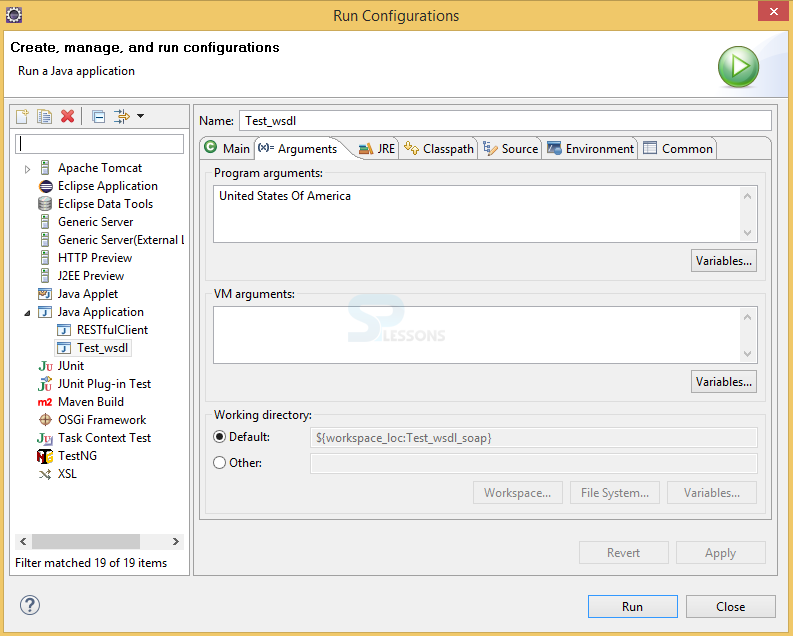
Now compile the code result will be as follows.
[java]<NewDataSet>
<Table>
<Country>British Indian Ocean Territory</Country>
<City>Diego Garcia</City>
</Table>
<Table>
<Country>India</Country>
<City>Ahmadabad</City>
</Table>
<Table>
<Country>India</Country>
<City>Akola</City>
</Table>
<Table>
<Country>India</Country>
<City>Aurangabad Chikalthan Aerodrome</City>
</Table>
<Table>
<Country>India</Country>
<City>Bombay / Santacruz</City>
</Table>
<Table>
<Country>India</Country>
<City>Bilaspur</City>
</Table>
<Table>
<Country>India</Country>
<City>Bhuj-Rudramata</City>
</Table>
<Table>
<Country>India</Country>
<City>Belgaum / Sambra</City>
</Table>
<Table>
<Country>India</Country>
<City>Bhopal / Bairagarh</City>
</Table>
<Table>
<Country>India</Country>
<City>Bhaunagar</City>
</Table>
<Table>
<Country>India</Country>
<City>Goa / Dabolim Airport</City>
</Table>
<Table>
<Country>India</Country>
<City>Indore</City>
</Table>
<Table>
<Country>India</Country>
<City>Jabalpur</City>
</Table>
<Table>
<Country>India</Country>
<City>Khandwa</City>
</Table>
<Table>
<Country>India</Country>
<City>Kolhapur</City>
</Table>
<Table>
<Country>India</Country>
<City>Nagpur Sonegaon</City>
</Table>
<Table>
<Country>India</Country>
<City>Rajkot</City>
</Table>
<Table>
<Country>India</Country>
<City>Sholapur</City>
</Table>
<Table>
<Country>India</Country>
<City>Agartala</City>
</Table>
<Table>
<Country>India</Country>
<City>Siliguri</City>
</Table>
<Table>
<Country>India</Country>
<City>Bhubaneswar</City>
</Table>
<Table>
<Country>India</Country>
<City>Calcutta / Dum Dum</City>
</Table>
<Table>
<Country>India</Country>
<City>Car Nicobar</City>
</Table>
<Table>
<Country>India</Country>
<City>Gorakhpur</City>
</Table>
<Table>
<Country>India</Country>
<City>Gauhati</City>
</Table>
<Table>
<Country>India</Country>
<City>Gaya</City>
</Table>
<Table>
<Country>India</Country>
<City>Imphal Tulihal</City>
</Table>
<Table>
<Country>India</Country>
<City>Jharsuguda</City>
</Table>
<Table>
<Country>India</Country>
<City>Jamshedpur</City>
</Table>
<Table>
<Country>India</Country>
<City>North Lakhimpur</City>
</Table>
<Table>
<Country>India</Country>
<City>Dibrugarh / Mohanbari</City>
</Table>
<Table>
<Country>India</Country>
<City>Port Blair</City>
</Table>
<Table>
<Country>India</Country>
<City>Patna</City>
</Table>
<Table>
<Country>India</Country>
<City>M. O. Ranchi</City>
</Table>
<Table>
<Country>India</Country>
<City>Agra</City>
</Table>
<Table>
<Country>India</Country>
<City>Allahabad / Bamhrauli</City>
</Table>
<Table>
<Country>India</Country>
<City>Amritsar</City>
</Table>
<Table>
<Country>India</Country>
<City>Varanasi / Babatpur</City>
</Table>
<Table>
<Country>India</Country>
<City>Bareilly</City>
</Table>
<Table>
<Country>India</Country>
<City>Kanpur / Chakeri</City>
</Table>
<Table>
<Country>India</Country>
<City>New Delhi / Safdarjung</City>
</Table>
<Table>
<Country>India</Country>
<City>New Delhi / Palam</City>
</Table>
<Table>
<Country>India</Country>
<City>Gwalior</City>
</Table>
<Table>
<Country>India</Country>
<City>Hissar</City>
</Table>
<Table>
<Country>India</Country>
<City>Jhansi</City>
</Table>
<Table>
<Country>India</Country>
<City>Jodhpur</City>
</Table>
<Table>
<Country>India</Country>
<City>Jaipur / Sanganer</City>
</Table>
<Table>
<Country>India</Country>
<City>Kota Aerodrome</City>
</Table>
<Table>
<Country>India</Country>
<City>Lucknow / Amausi</City>
</Table>
<Table>
<Country>India</Country>
<City>Satna</City>
</Table>
<Table>
<Country>India</Country>
<City>Udaipur Dabok</City>
</Table>
<Table>
<Country>India</Country>
<City>Bellary</City>
</Table>
<Table>
<Country>India</Country>
<City>Vijayawada / Gannavaram</City>
</Table>
<Table>
<Country>India</Country>
<City>Coimbatore / Peelamedu</City>
</Table>
<Table>
<Country>India</Country>
<City>Cochin / Willingdon</City>
</Table>
<Table>
<Country>India</Country>
<City>Cuddapah</City>
</Table>
<Table>
<Country>India</Country>
<City>Hyderabad Airport</City>
</Table>
<Table>
<Country>India</Country>
<City>Madurai</City>
</Table>
<Table>
<Country>India</Country>
<City>Mangalore / Bajpe</City>
</Table>
<Table>
<Country>India</Country>
<City>Madras / Minambakkam</City>
</Table>
<Table>
<Country>India</Country>
<City>Tiruchchirapalli</City>
</Table>
<Table>
<Country>India</Country>
<City>Thiruvananthapuram</City>
</Table>
<Table>
<Country>India</Country>
<City>Vellore</City>
</Table>
</NewDataSet>
[/java]