The LINQ to XML examples demonstrates the inserting the documents into the memory and saving the document in memory and deleting the document those examples are shown below.The code below demonstrates the reading of the XML document using LINQ is as shown.
[csharp]
using System;
using System.Collections.Generic;
using System.Linq;
using System.Xml.Linq;
namespace LINQtoXML
{
class ExampleOfXML
{
static void Main(string[] args)
{
string myXML = @"<Departments>
<Department>Finance</Department>
<Department>Sales</Department>
<Department>Max-Sales</Department>
<Department>Marketing</Department>
</Departments>";
XDocument xdoc = new XDocument();
xdoc = XDocument.Parse(myXML);
var result = xdoc.Element("Departments").Descendants();
foreach (XElement item in result)
{
Console.WriteLine("Department Name - " + item.Value);
}
Console.WriteLine("\nPress any key to continue.");
Console.ReadKey();
}
}
}
[/csharp]
Result
Compiling and execute the above code in a Visual Studio then user can get the output is a shown below.
The code below demonstrates the reading of the XML document using LINQ in VB
[csharp]
Imports System.Collections.Generic
Imports System.Linq
Imports System.Xml.Linq
Module Module1
Sub Main(ByVal args As String())
Dim myXML As String = "<Departments>" & vbCr & vbLf &
"<Department>Finance</Department>" & vbCr & vbLf &
"<Department>Sales</Department>" & vbCr & vbLf &
"<Department>Max-Sales</Department>" & vbCr & vbLf &
"<Department>Marketing</Department>" & vbCr & vbLf & "</Departments>"
Dim xdoc As New XDocument()
xdoc = XDocument.Parse(myXML)
Dim result = xdoc.Element("Departments").Descendants()
For Each item As XElement In result
Console.WriteLine("Department Name - " + item.Value)
Next
Console.WriteLine(vbLf & "Press any key to continue.")
Console.ReadKey()
End Sub
End Module
[/csharp]
Result
Compiling and execute the above code in a Visual Studio then user can get the output is a shown below.
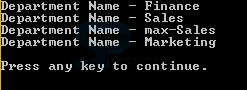
The code below demonstrates the adding new note to the XML document in C# is as shown.
[csharp]
using System;
using System.Collections.Generic;
using System.Linq;
using System.Xml.Linq;
namespace LINQtoXML
{
class ExampleOfXML
{
static void Main(string[] args)
{
string myXML = @"<Departments>
<Department>Finance</Department>
<Department>Sales</Department>
<Department>Max-Sales</Department>
<Department>Marketing</Department>
</Departments>";
XDocument xdoc = new XDocument();
xdoc = XDocument.Parse(myXML);
//Add new Element
xdoc.Element("Departments").Add(new XElement("Department", "Finance"));
//Add new Element at First
xdoc.Element("Departments").AddFirst(new XElement("Department", "Support"));
var result = xdoc.Element("Departments").Descendants();
foreach (XElement item in result)
{
Console.WriteLine("Department Name - " + item.Value);
}
Console.WriteLine("\nPress any key to continue.");
Console.ReadKey();
}
}
}
[/csharp]
Result
Compiling and execute the above code in a Visual Studio then user can get the output is a shown below.
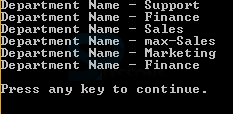
The code below demonstrates the adding new note to the XML document in VB is as shown.
[csharp]
Imports System.Collections.Generic
Imports System.Linq
Imports System.Xml.Linq
Module Module1
Sub Main(ByVal args As String())
Dim myXML As String = "<Departments>" & vbCr & vbLf &
"<Department>Finance</Department>" & vbCr & vbLf &
"<Department>Sales</Department>" & vbCr & vbLf &
"<Department>Max-Sales</Department>" & vbCr & vbLf &
"<Department>Marketing</Department>" & vbCr & vbLf &
"</Departments>"
Dim xdoc As New XDocument()
xdoc = XDocument.Parse(myXML)
xdoc.Element("Departments").Add(New XElement("Department", "Finance"))
xdoc.Element("Departments").AddFirst(New XElement("Department", "Support"))
Dim result = xdoc.Element("Departments").Descendants()
For Each item As XElement In result
Console.WriteLine("Department Name - " + item.Value)
Next
Console.WriteLine(vbLf & "Press any key to continue.")
Console.ReadKey()
End Sub
End Module
[/csharp]
Result
Compiling and execute the above code in a Visual Studio then user can get the output is a shown below.
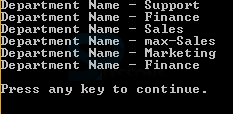
The code below demonstrates the Deleting a particular node from the XML Document which is shown in C# is as shown below.
[csharp]
using System;
using System.Collections.Generic;
using System.Linq;
using System.Xml.Linq;
namespace LINQtoXML
{
class ExampleOfXML
{
static void Main(string[] args)
{
string myXML = @"<Departments>
<Department>Support</Department>
<Department>Finance</Department>
<Department>Sales</Department>
<Department>Max-Sales</Department>
<Department>Marketing</Department>
<Department>Accounts</Department>
</Departments>";
XDocument xdoc = new XDocument();
xdoc = XDocument.Parse(myXML);
//Remove Sales Department
xdoc.Descendants().Where(s =>s.Value == "Sales").Remove();
var result = xdoc.Element("Departments").Descendants();
foreach (XElement item in result)
{
Console.WriteLine("Department Name - " + item.Value);
}
Console.WriteLine("\nPress any key to continue.");
Console.ReadKey();
}
}
}
[/csharp]
Result
Compiling and execute the above code in a Visual Studio then user can get the output is a shown below.
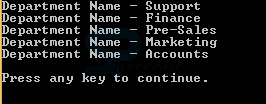
The code below demonstrates the Deleting a particular node from the XML Document which is shown in VB is as shown below.
[csharp]
Imports System.Collections.Generic
Imports System.Linq
Imports System.Xml.Linq
Module Module1
Sub Main(args As String())
Dim myXML As String = "<Departments>" & vbCr & vbLf &
"<Department>Support</Department>" & vbCr & vbLf &
"<Department>Finance</Department>" & vbCr & vbLf &
"<Department>Sales</Department>" & vbCr & vbLf &
"<Department>Max-Sales</Department>" & vbCr & vbLf &
"<Department>Marketing</Department>" & vbCr & vbLf &
"<Department>Accounts</Department>" & vbCr & vbLf & "</Departments>"
Dim xdoc As New XDocument()
xdoc = XDocument.Parse(myXML)
xdoc.Descendants().Where(Function(s) s.Value = "Sales").Remove()
Dim result = xdoc.Element("Departments").Descendants()
For Each item As XElement In result
Console.WriteLine("Department Name - " + item.Value)
Next
Console.WriteLine(vbLf & "Press any key to continue.")
Console.ReadKey()
End Sub
End Module
[/csharp]
Result
Compiling and execute the above code in a Visual Studio then user can get the output is a shown below.