The expression
"LINQ to Objects" means the utilisation of LINQ queries with
IEnumerable<T> accumulation directly i.e no need to use any middle LINQ supplier or API for example,
LINQ to SQL or
LINQ to XML. User can utilise LINQ to question or enumerable accumulations. In a fundamental sense, LINQ to Objects speaks to another way to deal with accumulations. In the old procedure, user need to compose complex foreach circles which predefined how to recover information from an accumulation.
LINQ to objects over a foreach loop have the many more advantages like powerful filtering, readability, capability of grouping and enhanced ordering with the minimal application coding. LINQ queries are no need to modify if need user can use with just little modifications. The code below demonstrates the LINQ to Object.
[csharp]
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LINQtoObjects
{
class Program
{
static void Main(string[] args)
{
string[] tools = { "Antony", "Dany", "Tony", "Cooper", "John",
"David" };
var list = from t in tools
select t;
StringBuilder sb = new StringBuilder();
foreach (string s in list)
{
sb.Append(s + Environment.NewLine);
}
Console.WriteLine(sb.ToString(), "Tools");
Console.ReadLine();
}
}
}
[/csharp]
Result
Compiling and execute the above code in a Visual Studio then user can get the output is a shown below.
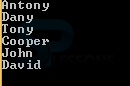
The code below demonstrates the querying a memory collection by using LINQ to the object is as shown below.
[csharp]
using System;
using System.Collections.Generic;
using System.Linq;
namespace LINQtoObjects
{
class Department
{
public int DepartmentId { get; set; }
public string Name { get; set; }
}
class LinqToObjects
{
static void Main(string[] args)
{
List<Department> departments = new List<Department>();
departments.Add(new Department { DepartmentId = 1, Name = "Finance" });
departments.Add(new Department { DepartmentId = 2, Name = "Sales" });
departments.Add(new Department { DepartmentId = 3, Name = "Marketing" });
var departmentList = from d in departments
select d;
foreach (var dept in departmentList)
{
Console.WriteLine("Department Id = {0} , Department Name = {1}",
dept.DepartmentId, dept.Name);
}
Console.WriteLine("\nPress any key to continue.");
Console.ReadKey();
}
}
}
[/csharp]
Result
Compiling and execute the above code in a Visual Studio then user can get the output is a shown below.

The code below demonstrates the querying a memory collection by using LINQ to the object in VB is as shown below.
[csharp]
Imports System.Collections.Generic
Imports System.Linq
Module Module1
Sub Main(ByVal args As String())
Dim account As New Department With {.Name = "Finance", .DepartmentId = 1}
Dim sales As New Department With {.Name = "Sales", .DepartmentId = 2}
Dim marketing As New Department With {.Name = "Marketing", .DepartmentId = 3}
Dim departments As New System.Collections.Generic.List(Of Department)(New Department() {account, sales, marketing})
Dim departmentList = From d In departments
For Each dept In departmentList
Console.WriteLine("Department Id = {0} , Department Name = {1}", dept.DepartmentId, dept.Name)
Next
Console.WriteLine(vbLf & "Press any key to continue.")
Console.ReadKey()
End Sub
Class Department
Public Property Name As String
Public Property DepartmentId As Integer
End Class
End Module
[/csharp]
Result
Compiling and execute the above code in a Visual Studio then user can get the output is a shown below.